在Android中,用户可以通过长按TextView来更改其值,要实现这个功能,我们需要使用`setOnLongClickListener`方法为TextView设置一个长按监听器,当用户长按TextView时,监听器会触发一个事件,我们可以在这个事件的处理函数中修改TextView的值。
以下是一个简单的示例:
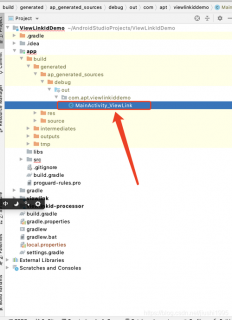
1. 在布局文件(如activity_main.xml)中添加一个TextView:
<TextView android:id="@+id/textView" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Hello World!" />
2. 然后,在Activity(如MainActivity.java)中为TextView设置长按监听器:
import androidx.appcompat.app.AppCompatActivity; import android.os.Bundle; import android.view.MotionEvent; import android.view.View; import android.widget.TextView; public class MainActivity extends AppCompatActivity { private TextView textView; private boolean isLongPressed = false; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); textView = findViewById(R.id.textView); textView.setOnTouchListener(new View.OnTouchListener() { @Override public boolean onTouch(View v, MotionEvent event) { switch (event.getAction()) { case MotionEvent.ACTION_DOWN: isLongPressed = false; break; case MotionEvent.ACTION_UP: isLongPressed = false; break; case MotionEvent.ACTION_MOVE: isLongPressed = false; break; case MotionEvent.ACTION_LONG_PRESS: isLongPressed = true; break; } return false; } }); } }
3. 接下来,我们需要在`onTouch`方法中判断用户是否长按了TextView,如果用户长按了TextView,我们可以修改其值:
if (isLongPressed) { textView.setText("New Value"); // 修改TextView的值为"New Value" } else { textView.setText("Hello World!"); // 恢复原始值"Hello World!" }
4. 将上述代码添加到`onTouch`方法中:
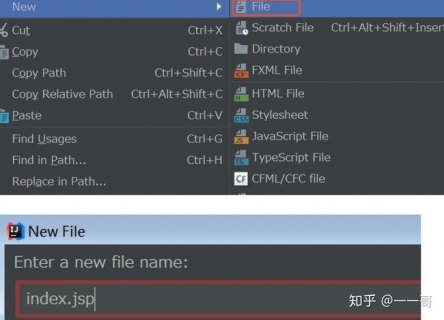
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); textView = findViewById(R.id.textView); textView.setOnTouchListener(new View.OnTouchListener() { @Override public boolean onTouch(View v, MotionEvent event) { switch (event.getAction()) { case MotionEvent.ACTION_DOWN: isLongPressed = false; break; case MotionEvent.ACTION_UP: isLongPressed = false; break; case MotionEvent.ACTION_MOVE: isLongPressed = false; break; case MotionEvent.ACTION_LONG_PRESS: isLongPressed = true; break; } if (isLongPressed) { textView.setText("New Value"); // 修改TextView的值为"New Value" } else { textView.setText("Hello World!"); // 恢复原始值"Hello World!" } return false; } }); }
当用户长按TextView时,其值会被更改为"New Value",松开手指后,值会恢复为原始值"Hello World!"。
滴滴空驶费,是对司机时间与油费的补偿,体现了对劳动者尊重,也是平台公平正义的体现。
遇到wifi密码正确却连不上网络的情况,可能是信号问题或是网络设置的小故障,别担心,尝试重启路由器或调整电脑的网络设置,通常能轻松解决问题。
笔记本关机响一声,不必过分忧心,或许是硬件的正常释放气息,关机后的响声,也许是它轻轻道别的旋律,给彼此一点理解,科技也有它的温度。
小米笔记本360度旋转设计实用又时尚,安装360软件更添便捷,两者结合,让工作和娱乐更加灵活高效。
别担心,T430i安装Windows10或许有些波折,但通过升级BIOS和驱动,定能顺利迎接新系统。